Android 12+ Deep Link Resolution
Some restrictions brought in by Google in Android 12 mean that deep linking or launching an activity from a BroadcastReceiver is no longer allowed. This functionality however is still retained for earlier versions of the Vibes Android SDK.
Vibes SDK Support for Deep Linking in Android 12
To be able to trigger the opening of any Activity after a user of an app with the Vibes SDK embedded opens a push notification, the following configuration changes need to be made in the AndroidManifest.xml file.
Such an activity must have these declared in its <intent-filters> -
- the action value set to vibes.action.push.OPENED .
- the category set to ${applicationId}
- a data entry declaring the URI pattern that it listens for. This is how each activity declares the specific data type it is interested in listening for.
This URI pattern must be included in the Deep Link portion of the push broadcasts, an example of which is shown below.
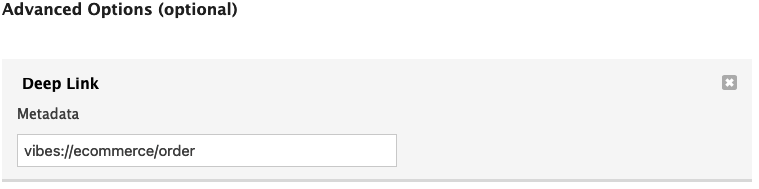
<activity
android:name=".views.OrderDetailActivity"
android:label="@string/title_activity_inbox_message"
android:theme="@style/AppTheme.NoActionBar"
android:parentActivityName=".views.LandingActivity">
<intent-filter>
<action android:name="com.vibes.action.push.OPENED" />
<category android:name="${applicationId}" />
<data android:scheme="vibes" android:host="ecommerce" android:path="/order" />
</intent-filter>
</activity>
In the example above, if we want to pass the orderId associated with that particular broadcast, we can pass this as part of custom properties.
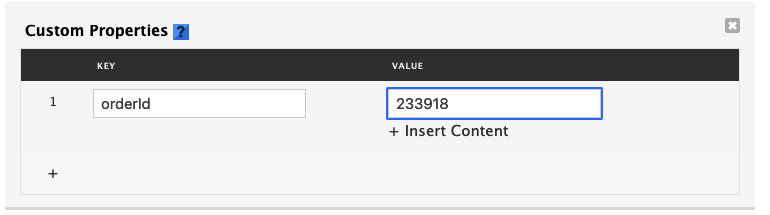
To access the push payload received within the activity, you will need to get the extras with the key Vibes.VIBES_REMOTE_MESSAGE_DATA. An example of such a usage is shown below.
protected void onCreate(Bundle savedInstanceState) {
...
Map\<String, String> pushMap = getIntent().getSerializableExtra(Vibes.VIBES_REMOTE_MESSAGE_DATA);
//this is for tracking which push messages have been opened by the user
Vibes.onPushMessageOpened(pushMap, context);
PushPayloadParser payload = new PushPayloadParser(pushMap);
try {
String orderId = payload.getCustomClientData().getString("orderId");
//fetch the order with the above orderId and then render the view.
} catch (JSONException e) {
e.printStackTrace();
}
....
}
Configuring event activity
By default, all clickthrough events will be received in the onCreate method of the MainActivity, unless an activity has been configured in the AndroidManifest.xml file which has the right configuration of <action>, <category> and <data> values.
For more on Android deep link resolution, please read here. For more on Android intents and intent filters, please read here.
Tracking Click of Push Notifications
Due to the Android 12 restrictions on BroadcastReveivers, developers will now be responsible for recording when users interact with a push message in their activities. This can be done by calling Vibes.onPushMessageOpened in your activity.
Map<String, String> pushMap = getIntent().getSerializableExtra(Vibes.VIBES_REMOTE_MESSAGE_DATA); //this is for tracking which push messages have been opened by the user Vibes.onPushMessageOpened(pushMap, context); PushPayloadParser payload = new PushPayloadParser(pushMap);
Updated 4 months ago